Copernicus Atmosphere Monitoring Service (CAMS)
Contents
Copernicus Atmosphere Monitoring Service (CAMS)¶
Global Fire Assimilation System (GFAS) data¶
The Copernicus Atmopshere Monitoring Service (CAMS) provides consistent and quality-controlled information related to air pollution and health and greenhouse gases. CAMS data consist of global forecasts and analyses
, global reanalyses
, fire emissions
and greenhouse gas flux inversions
.
This notebook provides an introduction to the CAMS Global Fire Assimilation System (GFAS) global fire emissions data. The CAMS Global Fire Assimilation System (GFAS) assimilates fire radiative power (FRP) observations from satellite-based sensors to produce daily estimates of wildfire and biomass burning emissions. It also provides information about injection heights derived from fire observations and meteorological information from the operational weather forecasts of ECMWF.
The GFAS data output includes spatially gridded Fire Radiative Power (FRP)
, dry matter burnt
and biomass burning emissions
for a large set of chemical, greenhouse gas and aerosol species. Data are available globally on a regular latitude-longitude grid with horizontal resolution of 0.1 degrees from 2003 to present.
Basic Facts
Spatial resolution: ~10km
Spatial coverage: Global
Time step: Daily data packaged in a monthly file
Data availability: since 2003
How to access the data
CAMS GFAS data are available in either GRIB
or netCDF
format. They can be downloaded from the Copernicus Atmosphere Data Store (ADS). You will need to create an ADS account here.
Get more information in the CAMS GFAS data documentation.
Load required libraries
import os
import xarray as xr
import numpy as np
import netCDF4 as nc
import pandas as pd
# Python libraries for visualization
%matplotlib inline
import matplotlib.pyplot as plt
import matplotlib.colors
from matplotlib.cm import get_cmap
from matplotlib.axes import Axes
import cartopy
import cartopy.crs as ccrs
from cartopy.mpl.gridliner import LONGITUDE_FORMATTER, LATITUDE_FORMATTER
from cartopy.mpl.geoaxes import GeoAxes
GeoAxes._pcolormesh_patched = Axes.pcolormesh
import warnings
warnings.simplefilter(action = "ignore", category = RuntimeWarning)
Load helper functions
%run ../functions.ipynb
Load and browse CAMS fire emissions data¶
Open a CAMS GFAS netCDF file with xarray
¶
CAMS GFAS fire emission data can be retrieved in either GRIB
or NetCDF
format. With the Python library xarray
and the open_dataset
function, we can easily read a single NetCDF
file.
CAMS GFAS fire emission data are three dimensional data, with the dimensions latitude
, longitude
and time
. The data file loaded has 31 time steps, from 1 August to 31 August 2021 and a global spatial coverage. The xarray dataset contains a data variable called frpfire
.
gfas_frpfire_xr = xr.open_dataset('../data/cams/gfas/frp/2021/08/_grib2netcdf-webmars-public-svc-green-007-6fe5cac1a363ec1525f54343b6cc9fd8-cGY9R_.nc')
gfas_frpfire_xr
<xarray.Dataset> Dimensions: (longitude: 3600, latitude: 1800, time: 31) Coordinates: * longitude (longitude) float32 0.05 0.15 0.25 0.35 ... 359.8 359.9 360.0 * latitude (latitude) float32 89.95 89.85 89.75 ... -89.75 -89.85 -89.95 * time (time) datetime64[ns] 2021-08-01 2021-08-02 ... 2021-08-31 Data variables: frpfire (time, latitude, longitude) float32 ... Attributes: Conventions: CF-1.6 history: 2022-02-15 11:56:28 GMT by grib_to_netcdf-2.24.0: grib_to_n...
- longitude: 3600
- latitude: 1800
- time: 31
- longitude(longitude)float320.05 0.15 0.25 ... 359.9 360.0
- units :
- degrees_east
- long_name :
- longitude
array([5.0000e-02, 1.5000e-01, 2.5000e-01, ..., 3.5975e+02, 3.5985e+02, 3.5995e+02], dtype=float32)
- latitude(latitude)float3289.95 89.85 89.75 ... -89.85 -89.95
- units :
- degrees_north
- long_name :
- latitude
array([ 89.95, 89.85, 89.75, ..., -89.75, -89.85, -89.95], dtype=float32)
- time(time)datetime64[ns]2021-08-01 ... 2021-08-31
- long_name :
- time
array(['2021-08-01T00:00:00.000000000', '2021-08-02T00:00:00.000000000', '2021-08-03T00:00:00.000000000', '2021-08-04T00:00:00.000000000', '2021-08-05T00:00:00.000000000', '2021-08-06T00:00:00.000000000', '2021-08-07T00:00:00.000000000', '2021-08-08T00:00:00.000000000', '2021-08-09T00:00:00.000000000', '2021-08-10T00:00:00.000000000', '2021-08-11T00:00:00.000000000', '2021-08-12T00:00:00.000000000', '2021-08-13T00:00:00.000000000', '2021-08-14T00:00:00.000000000', '2021-08-15T00:00:00.000000000', '2021-08-16T00:00:00.000000000', '2021-08-17T00:00:00.000000000', '2021-08-18T00:00:00.000000000', '2021-08-19T00:00:00.000000000', '2021-08-20T00:00:00.000000000', '2021-08-21T00:00:00.000000000', '2021-08-22T00:00:00.000000000', '2021-08-23T00:00:00.000000000', '2021-08-24T00:00:00.000000000', '2021-08-25T00:00:00.000000000', '2021-08-26T00:00:00.000000000', '2021-08-27T00:00:00.000000000', '2021-08-28T00:00:00.000000000', '2021-08-29T00:00:00.000000000', '2021-08-30T00:00:00.000000000', '2021-08-31T00:00:00.000000000'], dtype='datetime64[ns]')
- frpfire(time, latitude, longitude)float32...
- units :
- W m**-2
- long_name :
- Wildfire radiative power
[200880000 values with dtype=float32]
- Conventions :
- CF-1.6
- history :
- 2022-02-15 11:56:28 GMT by grib_to_netcdf-2.24.0: grib_to_netcdf /data/scratch/20220215-1150/2b/_mars-webmars-public-svc-green-000-6fe5cac1a363ec1525f54343b6cc9fd8-yo37HO.grib -o /data/scratch/20220215-1150/ac/_grib2netcdf-webmars-public-svc-green-007-6fe5cac1a363ec1525f54343b6cc9fd8-cGY9R_.nc -utime
Bring longitude coordinates onto a [-180,180] grid¶
The longitude values are on a [0,360] grid instead of a [-180,180] grid.
You can assign new values to coordinates in an xarray.Dataset
. You can do so with the assign_coords()
function, which you can apply onto a xarray.Dataset
. With the code below, you shift your longitude grid from [0,360] to [-180,180]. At the end, you sort the longitude values in an ascending order.
gfas_frpfire_xr_assigned = gfas_frpfire_xr.assign_coords(longitude=(((gfas_frpfire_xr.longitude + 180) % 360) - 180)).sortby('longitude')
gfas_frpfire_xr_assigned
<xarray.Dataset> Dimensions: (longitude: 3600, latitude: 1800, time: 31) Coordinates: * longitude (longitude) float32 -180.0 -179.9 -179.8 ... 179.8 179.9 180.0 * latitude (latitude) float32 89.95 89.85 89.75 ... -89.75 -89.85 -89.95 * time (time) datetime64[ns] 2021-08-01 2021-08-02 ... 2021-08-31 Data variables: frpfire (time, latitude, longitude) float32 ... Attributes: Conventions: CF-1.6 history: 2022-02-15 11:56:28 GMT by grib_to_netcdf-2.24.0: grib_to_n...
- longitude: 3600
- latitude: 1800
- time: 31
- longitude(longitude)float32-180.0 -179.9 ... 179.9 180.0
array([-179.95001, -179.85 , -179.75 , ..., 179.75 , 179.85 , 179.95001], dtype=float32)
- latitude(latitude)float3289.95 89.85 89.75 ... -89.85 -89.95
- units :
- degrees_north
- long_name :
- latitude
array([ 89.95, 89.85, 89.75, ..., -89.75, -89.85, -89.95], dtype=float32)
- time(time)datetime64[ns]2021-08-01 ... 2021-08-31
- long_name :
- time
array(['2021-08-01T00:00:00.000000000', '2021-08-02T00:00:00.000000000', '2021-08-03T00:00:00.000000000', '2021-08-04T00:00:00.000000000', '2021-08-05T00:00:00.000000000', '2021-08-06T00:00:00.000000000', '2021-08-07T00:00:00.000000000', '2021-08-08T00:00:00.000000000', '2021-08-09T00:00:00.000000000', '2021-08-10T00:00:00.000000000', '2021-08-11T00:00:00.000000000', '2021-08-12T00:00:00.000000000', '2021-08-13T00:00:00.000000000', '2021-08-14T00:00:00.000000000', '2021-08-15T00:00:00.000000000', '2021-08-16T00:00:00.000000000', '2021-08-17T00:00:00.000000000', '2021-08-18T00:00:00.000000000', '2021-08-19T00:00:00.000000000', '2021-08-20T00:00:00.000000000', '2021-08-21T00:00:00.000000000', '2021-08-22T00:00:00.000000000', '2021-08-23T00:00:00.000000000', '2021-08-24T00:00:00.000000000', '2021-08-25T00:00:00.000000000', '2021-08-26T00:00:00.000000000', '2021-08-27T00:00:00.000000000', '2021-08-28T00:00:00.000000000', '2021-08-29T00:00:00.000000000', '2021-08-30T00:00:00.000000000', '2021-08-31T00:00:00.000000000'], dtype='datetime64[ns]')
- frpfire(time, latitude, longitude)float32...
- units :
- W m**-2
- long_name :
- Wildfire radiative power
[200880000 values with dtype=float32]
- Conventions :
- CF-1.6
- history :
- 2022-02-15 11:56:28 GMT by grib_to_netcdf-2.24.0: grib_to_netcdf /data/scratch/20220215-1150/2b/_mars-webmars-public-svc-green-000-6fe5cac1a363ec1525f54343b6cc9fd8-yo37HO.grib -o /data/scratch/20220215-1150/ac/_grib2netcdf-webmars-public-svc-green-007-6fe5cac1a363ec1525f54343b6cc9fd8-cGY9R_.nc -utime
A quick check of the longitude coordinates of the new xarray.Dataset
shows you that the longitude values range now between [-180, 180].
gfas_frpfire_xr_assigned.longitude
<xarray.DataArray 'longitude' (longitude: 3600)> array([-179.95001, -179.85 , -179.75 , ..., 179.75 , 179.85 , 179.95001], dtype=float32) Coordinates: * longitude (longitude) float32 -180.0 -179.9 -179.8 ... 179.8 179.9 180.0
- longitude: 3600
- -180.0 -179.9 -179.8 -179.6 -179.5 ... 179.5 179.6 179.8 179.9 180.0
array([-179.95001, -179.85 , -179.75 , ..., 179.75 , 179.85 , 179.95001], dtype=float32)
- longitude(longitude)float32-180.0 -179.9 ... 179.9 180.0
array([-179.95001, -179.85 , -179.75 , ..., 179.75 , 179.85 , 179.95001], dtype=float32)
We can select the data variable with []
, which gives us access to the DataArray and more parameter attributes. Thus, the dataset values are wildfire radiative power
and the parameter unit is W m**-2
.
frpfire = gfas_frpfire_xr_assigned['frpfire']
frpfire
<xarray.DataArray 'frpfire' (time: 31, latitude: 1800, longitude: 3600)> [200880000 values with dtype=float32] Coordinates: * longitude (longitude) float32 -180.0 -179.9 -179.8 ... 179.8 179.9 180.0 * latitude (latitude) float32 89.95 89.85 89.75 ... -89.75 -89.85 -89.95 * time (time) datetime64[ns] 2021-08-01 2021-08-02 ... 2021-08-31 Attributes: units: W m**-2 long_name: Wildfire radiative power
- time: 31
- latitude: 1800
- longitude: 3600
- ...
[200880000 values with dtype=float32]
- longitude(longitude)float32-180.0 -179.9 ... 179.9 180.0
array([-179.95001, -179.85 , -179.75 , ..., 179.75 , 179.85 , 179.95001], dtype=float32)
- latitude(latitude)float3289.95 89.85 89.75 ... -89.85 -89.95
- units :
- degrees_north
- long_name :
- latitude
array([ 89.95, 89.85, 89.75, ..., -89.75, -89.85, -89.95], dtype=float32)
- time(time)datetime64[ns]2021-08-01 ... 2021-08-31
- long_name :
- time
array(['2021-08-01T00:00:00.000000000', '2021-08-02T00:00:00.000000000', '2021-08-03T00:00:00.000000000', '2021-08-04T00:00:00.000000000', '2021-08-05T00:00:00.000000000', '2021-08-06T00:00:00.000000000', '2021-08-07T00:00:00.000000000', '2021-08-08T00:00:00.000000000', '2021-08-09T00:00:00.000000000', '2021-08-10T00:00:00.000000000', '2021-08-11T00:00:00.000000000', '2021-08-12T00:00:00.000000000', '2021-08-13T00:00:00.000000000', '2021-08-14T00:00:00.000000000', '2021-08-15T00:00:00.000000000', '2021-08-16T00:00:00.000000000', '2021-08-17T00:00:00.000000000', '2021-08-18T00:00:00.000000000', '2021-08-19T00:00:00.000000000', '2021-08-20T00:00:00.000000000', '2021-08-21T00:00:00.000000000', '2021-08-22T00:00:00.000000000', '2021-08-23T00:00:00.000000000', '2021-08-24T00:00:00.000000000', '2021-08-25T00:00:00.000000000', '2021-08-26T00:00:00.000000000', '2021-08-27T00:00:00.000000000', '2021-08-28T00:00:00.000000000', '2021-08-29T00:00:00.000000000', '2021-08-30T00:00:00.000000000', '2021-08-31T00:00:00.000000000'], dtype='datetime64[ns]')
- units :
- W m**-2
- long_name :
- Wildfire radiative power
Create a geographical subset for Italy and Greece¶
Let’s subset our data to Italy and Greece.
For geographical subsetting, you can make use of the function generate_geographical_subset. You can use ?generate_geographical_subset
to open the docstring in order to see the function’s keyword arguments.
?generate_geographical_subset
Signature:
generate_geographical_subset(
xarray,
latmin,
latmax,
lonmin,
lonmax,
reassign=False,
)
Docstring:
Generates a geographical subset of a xarray.DataArray and if kwarg reassign=True, shifts the longitude grid
from a 0-360 to a -180 to 180 deg grid.
Parameters:
xarray(xarray.DataArray): a xarray DataArray with latitude and longitude coordinates
latmin, latmax, lonmin, lonmax(int): lat/lon boundaries of the geographical subset
reassign(boolean): default is False
Returns:
Geographical subset of a xarray.DataArray.
File: /tmp/ipykernel_8630/3979307327.py
Type: function
You can zoom into a region by specifying a bounding box
of interest. Let us set the extent to Italy and Greece with the following bounding box information:
lonmin=10
lonmax=30
latmin=35
latmax=45
Now, let us apply the function generate_geographical_subset to subset the frpfire
xarray.DataArray. Let us call the new xarray.DataArray frpfire_subset
.
frpfire_subset = generate_geographical_subset(xarray=frpfire,
latmin=latmin,
latmax=latmax,
lonmin=lonmin,
lonmax=lonmax)
frpfire_subset
<xarray.DataArray 'frpfire' (time: 31, latitude: 100, longitude: 200)> array([[[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., ... ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]], dtype=float32) Coordinates: * longitude (longitude) float32 10.05 10.15 10.25 10.35 ... 29.75 29.85 29.95 * latitude (latitude) float32 44.95 44.85 44.75 44.65 ... 35.25 35.15 35.05 * time (time) datetime64[ns] 2021-08-01 2021-08-02 ... 2021-08-31 Attributes: units: W m**-2 long_name: Wildfire radiative power
- time: 31
- latitude: 100
- longitude: 200
- 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0
array([[[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., ... ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], [[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]]], dtype=float32)
- longitude(longitude)float3210.05 10.15 10.25 ... 29.85 29.95
array([10.050003, 10.149994, 10.25 , 10.350006, 10.449997, 10.550003, 10.649994, 10.75 , 10.850006, 10.949997, 11.050003, 11.149994, 11.25 , 11.350006, 11.449997, 11.550003, 11.649994, 11.75 , 11.850006, 11.949997, 12.050003, 12.149994, 12.25 , 12.350006, 12.449997, 12.550003, 12.649994, 12.75 , 12.850006, 12.949997, 13.050003, 13.149994, 13.25 , 13.350006, 13.449997, 13.550003, 13.649994, 13.75 , 13.850006, 13.949997, 14.050003, 14.149994, 14.25 , 14.350006, 14.449997, 14.550003, 14.649994, 14.75 , 14.850006, 14.949997, 15.050003, 15.149994, 15.25 , 15.350006, 15.449997, 15.550003, 15.649994, 15.75 , 15.850006, 15.949997, 16.050003, 16.149994, 16.25 , 16.350006, 16.449997, 16.550003, 16.649994, 16.75 , 16.850006, 16.949997, 17.050003, 17.149994, 17.25 , 17.350006, 17.449997, 17.550003, 17.649994, 17.75 , 17.850006, 17.949997, 18.050003, 18.149994, 18.25 , 18.350006, 18.449997, 18.550003, 18.649994, 18.75 , 18.850006, 18.949997, 19.050003, 19.149994, 19.25 , 19.350006, 19.449997, 19.550003, 19.649994, 19.75 , 19.850006, 19.949997, 20.050003, 20.149994, 20.25 , 20.350006, 20.449997, 20.550003, 20.649994, 20.75 , 20.850006, 20.949997, 21.050003, 21.149994, 21.25 , 21.350006, 21.449997, 21.550003, 21.649994, 21.75 , 21.850006, 21.949997, 22.050003, 22.149994, 22.25 , 22.350006, 22.449997, 22.550003, 22.649994, 22.75 , 22.850006, 22.949997, 23.050003, 23.149994, 23.25 , 23.350006, 23.449997, 23.550003, 23.649994, 23.75 , 23.850006, 23.949997, 24.050003, 24.149994, 24.25 , 24.350006, 24.449997, 24.550003, 24.649994, 24.75 , 24.850006, 24.949997, 25.050003, 25.149994, 25.25 , 25.350006, 25.449997, 25.550003, 25.649994, 25.75 , 25.850006, 25.949997, 26.050003, 26.149994, 26.25 , 26.350006, 26.449997, 26.550003, 26.649994, 26.75 , 26.850006, 26.949997, 27.050003, 27.149994, 27.25 , 27.350006, 27.449997, 27.550003, 27.649994, 27.75 , 27.850006, 27.949997, 28.050003, 28.149994, 28.25 , 28.350006, 28.449997, 28.550003, 28.649994, 28.75 , 28.850006, 28.949997, 29.050003, 29.149994, 29.25 , 29.350006, 29.449997, 29.550003, 29.649994, 29.75 , 29.850006, 29.949997], dtype=float32)
- latitude(latitude)float3244.95 44.85 44.75 ... 35.15 35.05
- units :
- degrees_north
- long_name :
- latitude
array([44.95, 44.85, 44.75, 44.65, 44.55, 44.45, 44.35, 44.25, 44.15, 44.05, 43.95, 43.85, 43.75, 43.65, 43.55, 43.45, 43.35, 43.25, 43.15, 43.05, 42.95, 42.85, 42.75, 42.65, 42.55, 42.45, 42.35, 42.25, 42.15, 42.05, 41.95, 41.85, 41.75, 41.65, 41.55, 41.45, 41.35, 41.25, 41.15, 41.05, 40.95, 40.85, 40.75, 40.65, 40.55, 40.45, 40.35, 40.25, 40.15, 40.05, 39.95, 39.85, 39.75, 39.65, 39.55, 39.45, 39.35, 39.25, 39.15, 39.05, 38.95, 38.85, 38.75, 38.65, 38.55, 38.45, 38.35, 38.25, 38.15, 38.05, 37.95, 37.85, 37.75, 37.65, 37.55, 37.45, 37.35, 37.25, 37.15, 37.05, 36.95, 36.85, 36.75, 36.65, 36.55, 36.45, 36.35, 36.25, 36.15, 36.05, 35.95, 35.85, 35.75, 35.65, 35.55, 35.45, 35.35, 35.25, 35.15, 35.05], dtype=float32)
- time(time)datetime64[ns]2021-08-01 ... 2021-08-31
- long_name :
- time
array(['2021-08-01T00:00:00.000000000', '2021-08-02T00:00:00.000000000', '2021-08-03T00:00:00.000000000', '2021-08-04T00:00:00.000000000', '2021-08-05T00:00:00.000000000', '2021-08-06T00:00:00.000000000', '2021-08-07T00:00:00.000000000', '2021-08-08T00:00:00.000000000', '2021-08-09T00:00:00.000000000', '2021-08-10T00:00:00.000000000', '2021-08-11T00:00:00.000000000', '2021-08-12T00:00:00.000000000', '2021-08-13T00:00:00.000000000', '2021-08-14T00:00:00.000000000', '2021-08-15T00:00:00.000000000', '2021-08-16T00:00:00.000000000', '2021-08-17T00:00:00.000000000', '2021-08-18T00:00:00.000000000', '2021-08-19T00:00:00.000000000', '2021-08-20T00:00:00.000000000', '2021-08-21T00:00:00.000000000', '2021-08-22T00:00:00.000000000', '2021-08-23T00:00:00.000000000', '2021-08-24T00:00:00.000000000', '2021-08-25T00:00:00.000000000', '2021-08-26T00:00:00.000000000', '2021-08-27T00:00:00.000000000', '2021-08-28T00:00:00.000000000', '2021-08-29T00:00:00.000000000', '2021-08-30T00:00:00.000000000', '2021-08-31T00:00:00.000000000'], dtype='datetime64[ns]')
- units :
- W m**-2
- long_name :
- Wildfire radiative power
Above, you see that the variable frpfire_subset
has two attributes, units
and long_name
. Let us define variables for those attributes. The variables can be used for visualizing the data.
units = frpfire_subset.units
long_name= frpfire_subset.long_name
Visualise CAMS GFAS fire emissions data¶
You can use the function visualize_pcolormesh to visualize the CAMS global fire emissions data with matplotlib’s function pcolormesh
.
You can make use of the data attributes units
and long_name
and use them for plotting.
visualize_pcolormesh(data_array=frpfire_subset.isel(time=4).data,
longitude=frpfire_subset.longitude.data,
latitude=frpfire_subset.latitude.data,
projection=ccrs.PlateCarree(),
color_scale='YlOrRd',
unit=units,
long_name=long_name + ' ' + str(frpfire_subset.isel(time=4).time.data)[0:10],
vmin=0,
vmax=1,
lonmin=lonmin,
lonmax=lonmax,
latmin=latmin,
latmax=latmax,
set_global=False)
(<Figure size 1440x720 with 2 Axes>,
<GeoAxesSubplot:title={'center':'Wildfire radiative power 2021-08-05'}>)
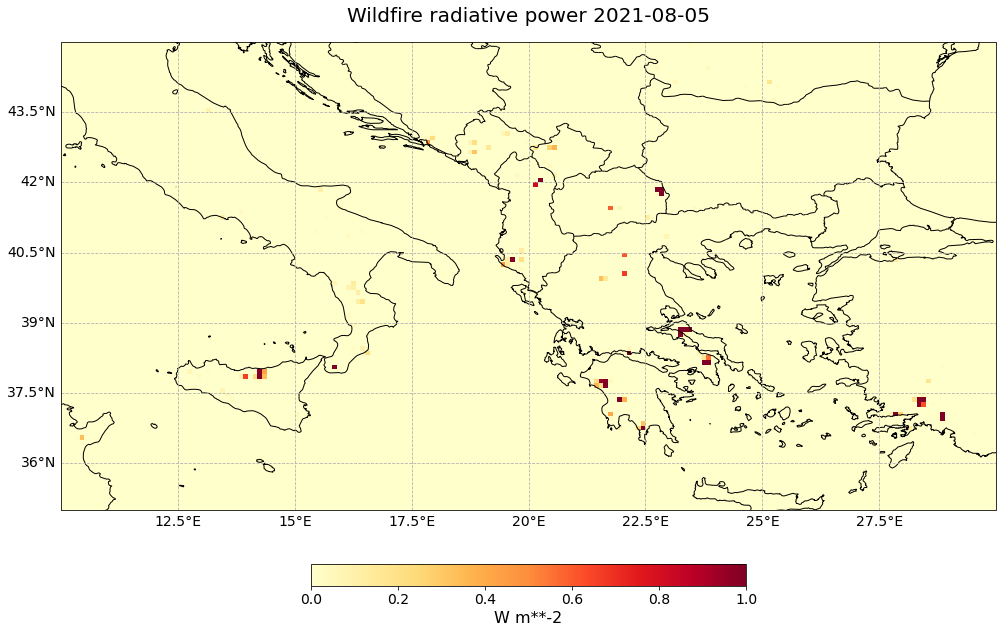
References¶
Kaiser, J. W., and Coauthors. (2012). Biomass burning emissions estimated with a global fire assimilation system based on observed fire radiative power, Biogeosciences, 9, 527-554, https://doi.org/10.5194/bg-9-527-2012.
Some code in this notebook was adapted from the following source:
copyright: 2022, EUMETSAT
license: MIT
retrieved: 2022-06-28 by Sabrina Szeto
Return to the case study
Monitoring active fires with next-generation satellites: Mediterranean Fires Case Study
Fire Radiative Power (CAMS GFAS)